C Program Code Solutions
Enhance your skills with real-world coding challenges and comprehensive word problem solutions for effective learning.
Problem 1 : Reverse a String
Problem Statement: 1. Write a program to reverse a string entered by the user.
Example Input: "hello"
Example Output: "olleh"
Solution:
Strings in C are arrays of characters ending with a null character \0.
To reverse a string, we can swap the first character with the last, the second with the second last, and so on.
Use two pointers: one starting from the beginning and one from the end. Swap characters at these pointers until they meet in the middle.
Code:
Problem 2 : Palindrome Number Check
Problem Statement: Check if a given number is a palindrome.
Example Input: 121
Example Output: Palindrome
Solution:
A number is a palindrome if it reads the same backward and forward.
To check this, reverse the number and compare it with the original.
Use modulus % to extract digits and build the reversed number.
Code:
Problem 3 : Find GCD of Two Numbers
Problem Statement: Write a program to find the greatest common divisor (GCD) of two numbers.
Example Input: 36, 60
Example Output: 12
Solution:
The GCD of two numbers is the largest number that divides both numbers without a remainder.
Use the Euclidean Algorithm:
Subtract the smaller number from the larger repeatedly until one number becomes 0.
The non-zero number at the end is the GCD.
Code:
Problem 4 : Sum of Digits in a Number
Problem Statement: Write a program to calculate the sum of digits in a number.
Example Input: 1234
Example Output: 10
Solution:
Extract the last digit using modulus %.
Add it to a sum variable.
Remove the last digit using integer division / and repeat the process until the number becomes 0.
Code:
Problem 5 : Fibonacci Series
Problem Statement: Print the first n terms of the Fibonacci series.
Example Input: 5
Example Output: 0 1 1 2 3
Solution:
The Fibonacci series is a sequence where each term is the sum of the two preceding ones.
Start with 0 and 1.
Use a loop to generate subsequent terms by adding the previous two.
Code:
Problem 6: Count Vowels in a String
Problem Statement: Write a program to count the number of vowels in a string.
Example Input: "education"
Example Output: 5
Solution:
Loop through the string character by character.
Check if each character is a vowel (a, e, i, o, u) either in lowercase or uppercase.
Code:
Problem 7: Find the Second Largest Element in an Array
Problem Statement: Write a program to find the second largest element in an array.
Example Input: {12, 35, 1, 10, 34, 1}
Example Output: 34
Solution:
Traverse the array once to find the largest element.
Traverse again to find the largest element smaller than the first largest (i.e., the second largest).
Code:
Problem 8: Employee Salary Slip Generator
Problem Statement: Write a program to calculate the gross salary of an employee. The gross salary is calculated as:
Gross Salary = Basic + HRA + DA
Where:HRA is 20% of Basic Salary
DA is 80% of Basic Salary
Example Input:
Basic Salary: 25000
Example Output:
HRA: 5000, DA: 20000, Gross Salary: 50000
Solution:
To calculate the gross salary, compute HRA as 20% and DA as 80% of the basic salary.
Use simple arithmetic operations to compute the final value.
Code:
Problem 9 : Electricity Bill Calculator
Problem Statement: Write a program to calculate the electricity bill based on the following tariff:
First 100 units: ₹1.5/unit
Next 200 units: ₹2/unit
Above 300 units: ₹3/unit
Example Input:
Units Consumed: 350
Example Output:
Bill Amount: ₹650
Solution:
Use conditional checks to apply the correct rate for each slab.
Compute the cost for units in each slab and sum them up.
Code:
Problem 10: Student Grade Calculator
Problem Statement: Write a program to calculate the grade of a student based on their marks:
Marks >= 90: Grade A
Marks >= 80 and <90: Grade B
Marks >= 70 and <80: Grade C
Marks >= 60 and <70: Grade D
Marks < 60: Grade F
Example Input:
Marks: 85
Example Output:
Grade: B
Solution:
Use conditional statements to evaluate the range in which the marks fall.
Assign the corresponding grade based on the range.
Code:
Problem 11: Currency Denomination Calculator
Problem Statement: Write a program to calculate the minimum number of notes required for a given amount. Consider the denominations: ₹2000, ₹500, ₹200, ₹100, ₹50, ₹20, ₹10, ₹5, ₹1.
Example Input:
Amount: 2673
Example Output:
₹2000: 1, ₹500: 1, ₹100: 1, ₹50: 1, ₹20: 1, ₹1: 3
Solution:
Start with the largest denomination and calculate the number of notes by dividing the amount by the denomination.
Reduce the amount by the value of these notes and repeat for the next smaller denomination.
Code:
Problem 12: Banking System - Simple Interest Calculator
Problem Statement: Write a program to calculate the simple interest for a loan. The formula is:
Simple Interest (SI) = (Principal Rate Time) / 100
Example Input:
Principal: 10000
Rate: 5
Time: 2
Example Output:
Simple Interest: ₹1000
Solution:
Use the formula directly for computation.
Read the principal amount, rate of interest, and time from the user.
Code:
Problem 13: Voting Eligibility Checker
Problem Statement: Write a program to check if a person is eligible to vote.
Eligibility Criteria: Age must be 18 or above.
Example Input:
Age: 16
Example Output:
Not Eligible to Vote
Solution:
Use a conditional statement to check if the age is 18 or greater.
Code:
Problem 14: Factorial of a Number
Problem Statement: Write a program to calculate the factorial of a number.
Example Input:
Number: 5
Example Output:
Factorial: 120
Solution:
Factorial of a number is the product of all integers from 1 to that number.
Use a loop to calculate the product iteratively.
Code:
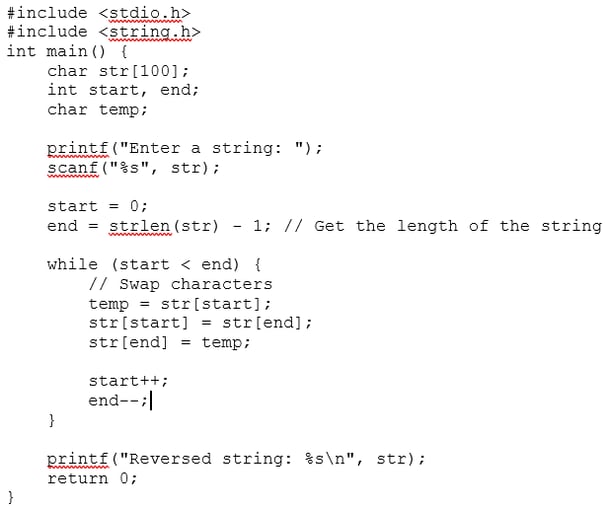
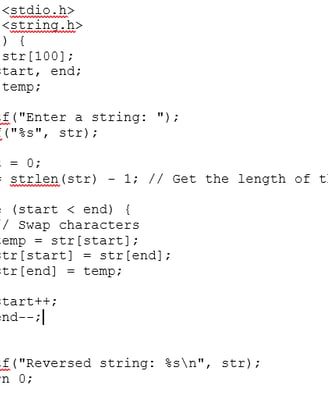
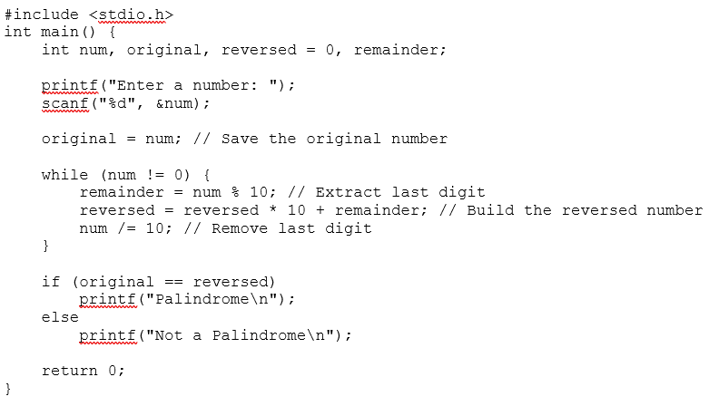
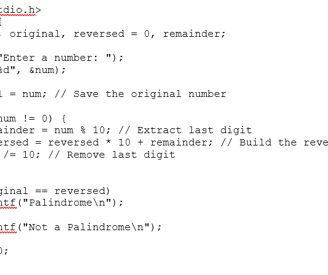
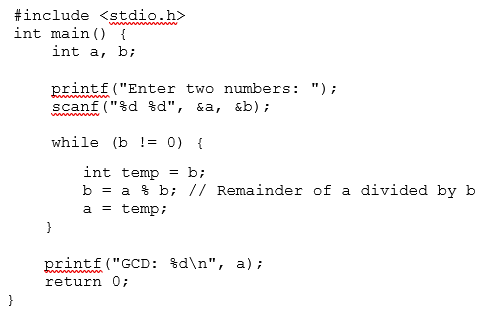
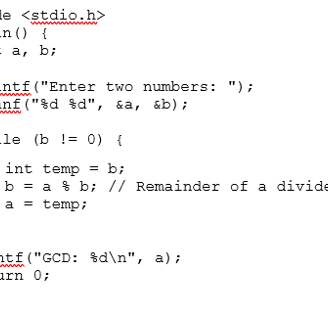
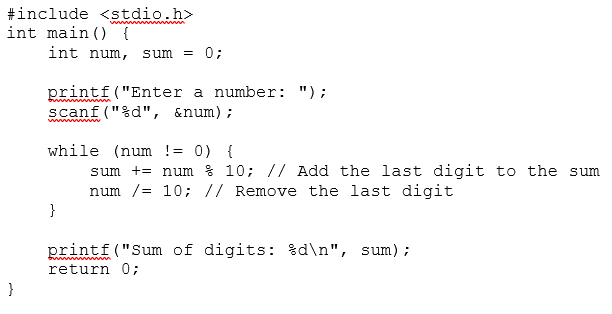
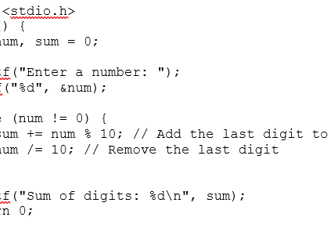
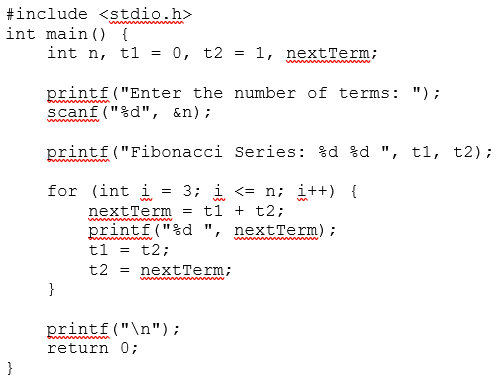
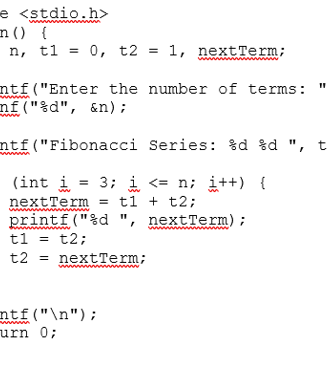
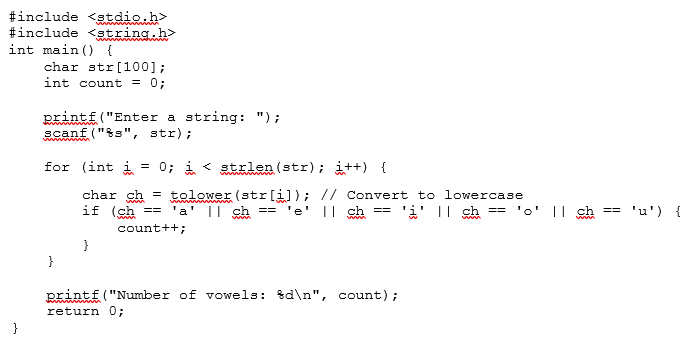
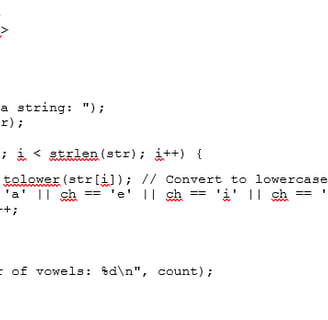
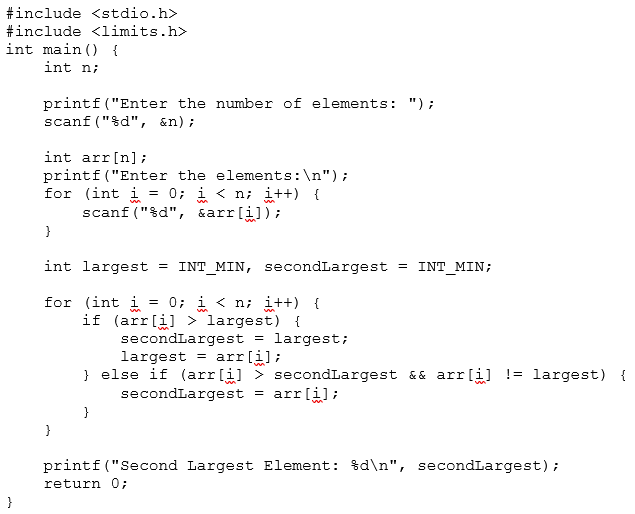
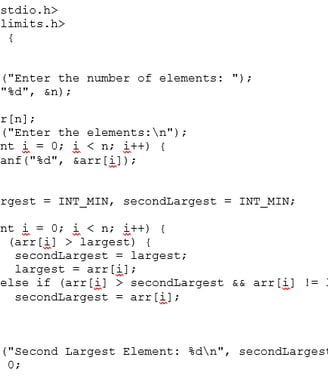
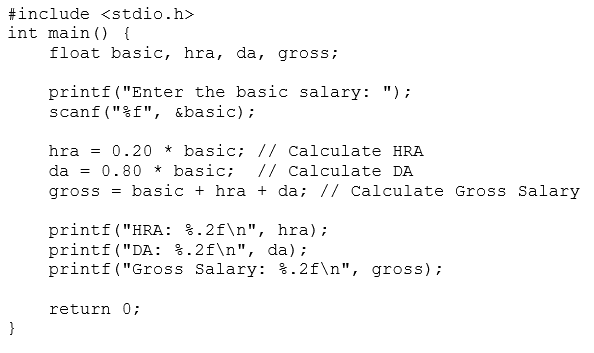
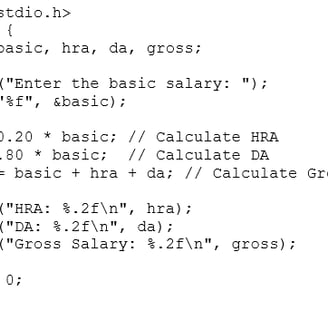
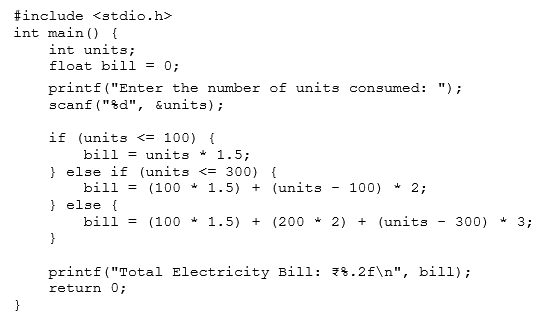
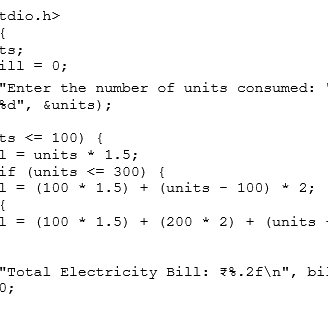
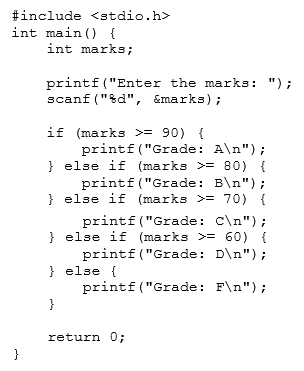
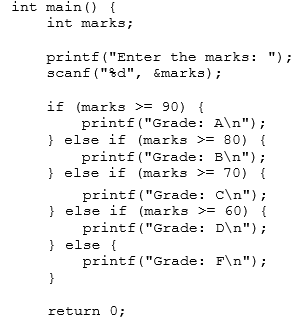
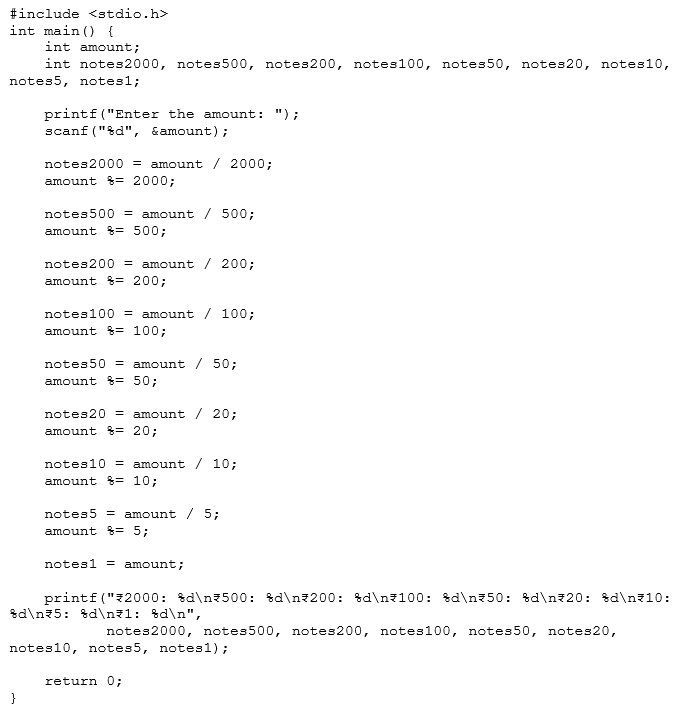
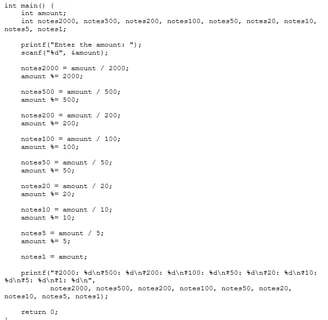
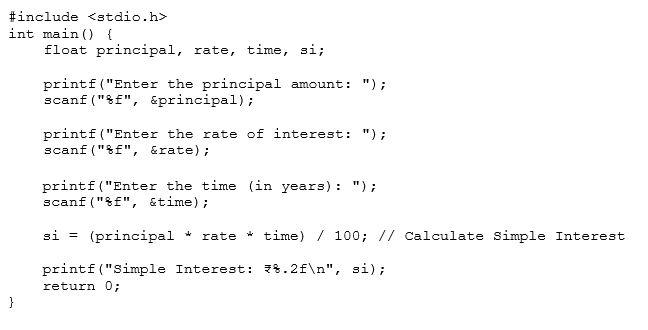
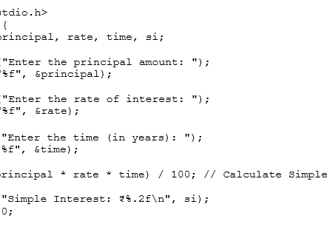
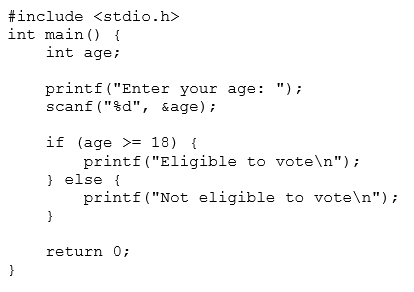
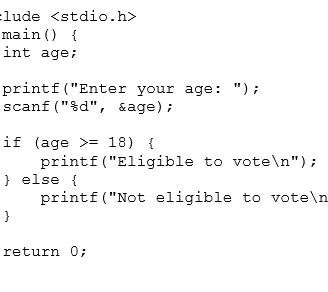
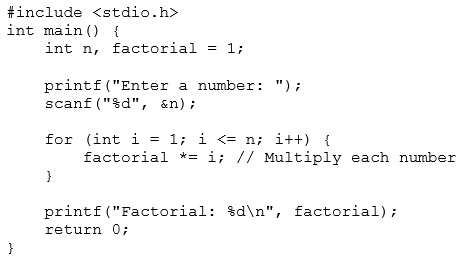
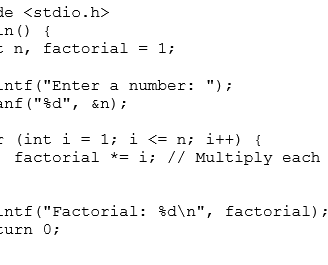
© 2024. All rights reserved.
Professor of Practice
Bridging academia and industry through innovative teaching and real-world experience for academic success.